|
Getting Started with Ch Control System Toolkit
To help users
to get familiar with Ch Control System Toolkit,
a sample program will be used to illustrate
basic features and applications of Ch Control
System Toolkit.
In this example, the control system shown below
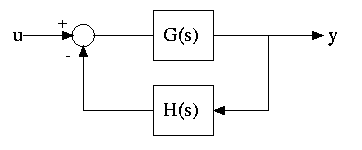
consists of a plant and a feedback controller with transfer functions
3
G(s) = ------
s(s+2)
2
H(s) = ---
3
The closed-loop transfer function of the system is
G(s)
T(s) = ----------
1+G(s)H(s)
3
= --------
s^2+2s+2
The step response of the closed-loop system
will be plotted in this example.
The code listed below is a Ch program
using Ch Control System Toolkit for solving this problem.
#include
int main() {
double num[1] = {3};
double den[3] = {1, 2, 2};
class CPlot plot;
class CControl sys;
sys.model("tf", num, den);
sys.step(&plot, NULL, NULL, NULL);
return 0;
}
The first line of the program
#include
includes the header file control.h
which defines the class CControl, macros, and prototypes
of member functions. Like a C/C++ program, a Ch program
will start to execute at the main() function
after the program is parsed. The next two lines
double num[1] = {3};
double den[3] = {1, 2, 2};
define two arrays num and den
to store the coefficients of the
numerator and denominator of the polynomials of the
transfer function T(s), respectively. Line
class CPlot plot;
defines a class CPlot for creating and
manipulating two and three dimensional plotting.
The CPlot class is defined in header file chplot.h
which is included in control.h header file.
Line
class CControl sys;
instantiates a CControl class.
Line
sys.model("tf", num, den);
constructs a transfer function model of the system.
The type of models created by
member function
model() is specified by
the first argument. For example, string "tf"
indicates transfer function model. The second and
third arguments specify the coefficients of the
numerator and denominator polynomials of the transfer function.
Ch Control System Toolkit supports
transfer function (TF), zero-pole-gain (ZPK),
state-space (SS), and other LTI models.
The details of model types supported by
Ch Control System Toolkit are
described in Ch Control System Toolkit User's Guide.
Like C++, the keyword class is optional in Ch.
Line
sys.step(&plot, NULL, NULL, NULL);
computes and plots the step response of the system.
Member function step() has four arguments.
The first argument is a pointer to an existing object
of class CPlot. The other three arguments are
arrays of reference containing the output of the step
response, time vector, and state trajectories.
If the output data are not required, these three
arguments can be set to NULL. The step
response of the system, when
the above program is executed, is shown in the figure below.
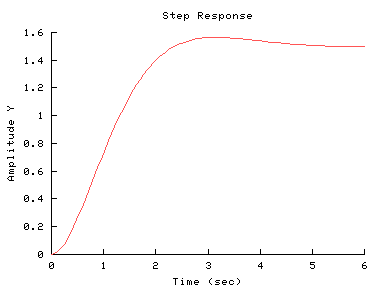
As another example, for a control system represted by transfer function
2s^2-3.5s+1.5
T(s) = --------------
s^2-1.5s+0.9
The following program can generate the root locus of the system modeled
in discrete form as shown in the plot.
#include // Ch Control System Toolkit header file
int main() {
// numerator of the transfer function
double num[3] = {2, -3.5, 1.5};
// denominator of the transfer function
double den[3] = {1, -1.5, 0.9};
CPlot plot; // plotting class
CControl sys; // control class
/* build control system in transfer function in discrete model */
sys.model("tf", num, den, -1);
sys.zgrid(1); // use z-grid
sys.rlocus(&plot, NULL, NULL);// display root locus
return 0;
}
More application examples in comparison with
MATLAB Control System Toolbox
can be found
here.
|